Mixin Design Pattern
Additional Patterns: Mixin Design Pattern
What is a Mixin in JavaScript?
View Answer:
Here's an example of a Mixin in JavaScript:
let sayMixin = {
sayHi() {
console.log(`Hello ${this.name}`);
},
sayBye() {
console.log(`Bye ${this.name}`);
}
};
class User {
constructor(name) {
this.name = name;
}
}
// Copy the methods
Object.assign(User.prototype, sayMixin);
// Now User can say Hi and Bye
let user = new User("John");
user.sayHi(); // Output: Hello John
user.sayBye(); // Output: Bye John
In this example, the sayMixin
object contains two methods: sayHi
and sayBye
. These methods are copied into the User
class using Object.assign
, so now instances of User
can use the sayHi
and sayBye
methods. This demonstrates the use of a Mixin to "mix in" functionality from another object.
What is the Mixin design pattern in JavaScript?
View Answer:
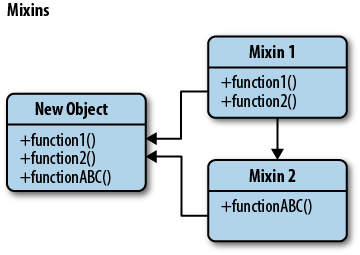
// ES2015+ keywords/syntax used: class, constructor, const
class Person {
constructor(firstName, lastName) {
this.firstName = firstName;
this.lastName = lastName;
this.gender = 'male';
}
}
// a new instance of Person can then easily be created as follows:
const clark = new Person('Clark', 'Kent');
// ES2015+ keywords/syntax used: class, constructor, extends super, const
class Superhero extends Person {
constructor(firstName, lastName, powers) {
// Invoke the superclass constructor
super(firstName, lastName);
this.powers = powers;
}
}
// A new instance of Superher gets created as follows
const SuperMan = new Superhero('Clark', 'Kent', ['flight', 'heat-vision']);
console.log(SuperMan);
/* Outputs Person attributes as well as powers
Superhero {
firstName: 'Clark',
lastName: 'Kent',
gender: 'male',
powers: [ 'flight', 'heat-vision' ]
}
*/
// ES2015+ keywords/syntax used: class, constructor, extends, const, arrow functions
// Define a simple Car constructor
class Car {
constructor({ model, color }) {
this.model = model || 'no model provided';
this.color = color || 'no colour provided';
}
}
// Mixin
const Mixin = (superclass) =>
class extends superclass {
driveForward() {
console.log('drive forward');
}
driveBackward() {
console.log('drive backward');
}
driveSideways() {
console.log('drive sideways');
}
};
class MyCar extends Mixin(Car) {}
// Create a new Car
const myCar = new MyCar({
model: 'Ford Escort',
color: 'blue',
});
// Test to make sure we now have access to the methods
myCar.driveForward();
myCar.driveBackward();
// Outputs:
// drive forward
// drive backward
const mySportsCar = new MyCar({
model: 'Porsche',
color: 'red',
});
mySportsCar.driveSideways();
// Outputs:
// drive sideways
To which design pattern family does the Mixin pattern belong?
View Answer:
Why are Mixins used in JavaScript?
View Answer:
When should you utilize the JavaScript Mixin Pattern?
View Answer:
What are some of the benefits of using the Mixin pattern?
View Answer:
Some of the benefits of using the Mixin pattern are:
- It reduces code duplication and increases reusability.
- It allows adding multiple behaviors to a class without inheritance.
- It provides flexibility and modularity for composing classes.
- It avoids conflicts with existing class methods by using proper naming conventions.
What is the main drawback of Mixins?
View Answer:
What problem does the Mixin pattern solve?
View Answer:
// Define the mixin
let swimMixin = {
swim: function() {
return "I can swim!";
}
};
// Define an object
let duck = {
name: 'Donald',
age: 5
};
// Apply the mixin to the object
Object.assign(duck, swimMixin);
console.log(duck.swim()); // Outputs: "I can swim!"
In this example, the swimMixin
is a mixin that defines a swim
function. The Object.assign
function is used to mix the swimMixin
into the duck
object, effectively giving the duck
the ability to swim
.
What are the main components of the Mixin pattern?
View Answer:
The main components of the Mixin pattern in modern JavaScript are:
Base objects or classes, from which we want to borrow methods or properties.
A Mixin function, that combines the properties and methods of the base objects or classes into a new object or class.
Target Object: The target object is the object that will receive the mixed-in functionality
Here's a simple example:
// Base objects (or classes)
const CanWalk = {
walk() {
console.log('Walking...');
}
}
const CanSwim = {
swim() {
console.log('Swimming...');
}
}
// Mixin function
function mixin(target, ...sources) {
Object.assign(target, ...sources);
}
// Usage - duck is our target object
const duck = {};
mixin(duck, CanWalk, CanSwim);
duck.walk(); // Output: 'Walking...'
duck.swim(); // Output: 'Swimming...'
In this example:
CanWalk
andCanSwim
are the base objects. They have thewalk
andswim
methods respectively.mixin
is the Mixin function. It takes a target object and one or more source objects, and it uses theObject.assign
function to copy the properties of the source objects into the target object.duck
is the target object. After themixin
function is called withduck
,CanWalk
, andCanSwim
, theduck
object has both thewalk
andswim
methods.
Note that the Mixin pattern can be implemented with both objects and classes in JavaScript. In the example above, objects are used for simplicity.
How does the Mixin pattern differ from inheritance?
View Answer:
How do Mixins differ from traditional inheritance?
View Answer:
How can we avoid naming conflicts in Mixins?
View Answer:
Can Mixins be used with ES6 classes?
View Answer:
// Define the Mixins
const Walkable = (Base) => class extends Base {
walk() {
console.log('Walking...');
}
};
const Swimable = (Base) => class extends Base {
swim() {
console.log('Swimming...');
}
};
// Define the base class
class Creature {}
// Create a new class using the Mixins
class Frog extends Swimable(Walkable(Creature)) {
jump() {
console.log('Jumping...');
}
}
// Instantiate the new class
const frog = new Frog();
frog.walk(); // Output: 'Walking...'
frog.swim(); // Output: 'Swimming...'
frog.jump(); // Output: 'Jumping...'
In this example, Walkable
and Swimable
are Mixins that can be used to extend a base class with walk
and swim
methods, respectively. They're implemented as functions that take a base class as a parameter and return a new class that extends the base class with the additional methods.
The Frog
class is created by applying the Swimable
and Walkable
Mixins to the Creature
base class. The resulting class has walk
, swim
, and jump
methods.
Note that the order in which the Mixins are applied matters. If a Mixin overrides a method provided by a Mixin that was applied earlier, the last applied Mixin's method will be the one used.
Do Mixins support private properties or methods?
View Answer:
// Define a Mixin that uses a private method and a public method to interact with it
const Walkable = (Base) => class extends Base {
#distance = 0;
walk() {
this.#distance++;
this.#logDistance();
}
#logDistance() {
console.log(`Walked ${this.#distance} step(s)`);
}
};
// Define a base class
class Creature {}
// Create a new class using the Mixin
class Human extends Walkable(Creature) {}
// Instantiate the new class
const human = new Human();
human.walk(); // Output: 'Walked 1 step(s)'
human.walk(); // Output: 'Walked 2 step(s)'
In this example, Walkable
is a mixin that introduces a private field #distance
and a private method #logDistance
into any class it's mixed into. #logDistance
is truly private and can't be called from an instance of Human
. It's used internally by the walk
method, which is publicly available and increments the #distance
field each time it's called. The use of private fields and methods encapsulates and protects the internal state and behavior of the mixin.
Can a class use multiple Mixins?
View Answer:
// Define some simple mixins
const Walkable = Base => class extends Base {
walk() {
console.log("Walking...");
}
};
const Swimable = Base => class extends Base {
swim() {
console.log("Swimming...");
}
};
const Flyable = Base => class extends Base {
fly() {
console.log("Flying...");
}
};
// Base class
class Animal {}
// Use multiple mixins with a class
class Duck extends Flyable(Swimable(Walkable(Animal))) {}
// Instantiate and use the mixed class
const daffy = new Duck();
daffy.walk(); // Output: "Walking..."
daffy.swim(); // Output: "Swimming..."
daffy.fly(); // Output: "Flying..."
In this example, the Duck
class is created by applying the Flyable
, Swimable
, and Walkable
mixins to the Animal
base class. The order in which the mixins are applied can be important if the mixins provide methods with the same name, as the mixin applied last will override methods from the earlier mixins.
Are Mixins commonly used in JavaScript frameworks?
View Answer:
Here is an example of using mixins in Vue.js:
// Define a mixin object
var myMixin = {
created: function () {
this.hello()
},
methods: {
hello: function () {
console.log('Hello from mixin!')
}
}
}
// Define a component that uses this mixin
var Component = Vue.extend({
mixins: [myMixin]
})
var component = new Component() // Logs "Hello from mixin!" when created
In this example, we've defined a mixin myMixin
that has a lifecycle hook created
and a method hello
. When the Component
that uses this mixin is created, it will automatically call the hello
method and log the message "Hello from mixin!".
As for React, it doesn't natively support mixins, but similar functionality can be achieved using higher-order components (HOCs) or hooks. Here is an example of a React Hook:
// Define a custom hook
function useHello() {
React.useEffect(() => {
console.log('Hello from hook!');
}, []);
// Other logic here...
}
// Use the custom hook in a component
function MyComponent() {
useHello();
return <h1>Hello World</h1>;
}
In this React example, the useHello
hook logs "Hello from hook!" when the MyComponent
is rendered. Hooks are a way to reuse stateful logic, not state itself, across components.