Event loop - Microtasks/Macrotasks
Miscellaneous: Event loop
What is the Event Loop in JavaScript?
View Answer:
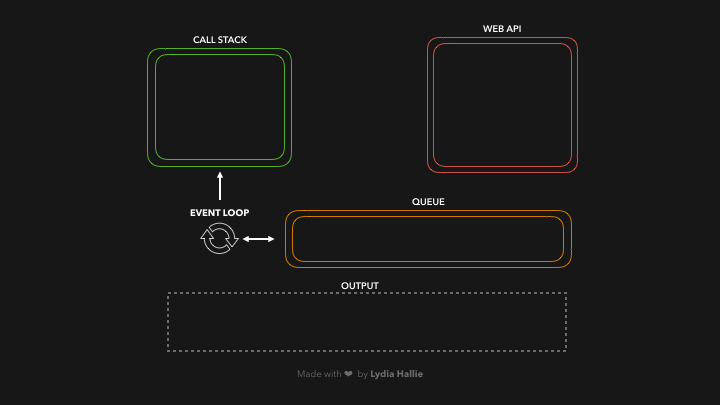
How are the tasks from the queue processed or handled?
View Answer:
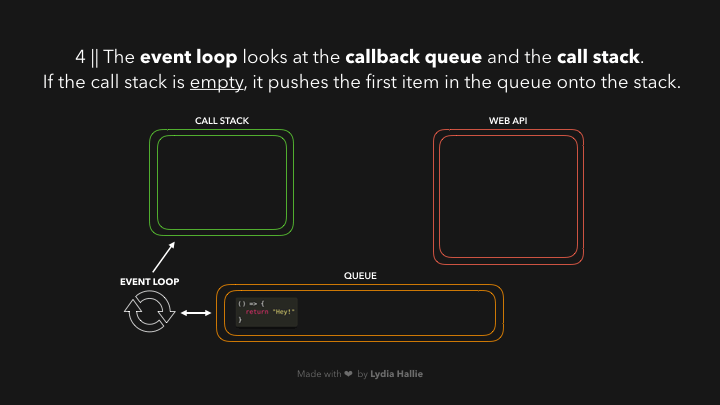
What is the best method to avoid difficulties caused by CPU-intensive tasks?
View Answer:
let i = 0;
let start = Date.now();
function count() {
// do a piece of the heavy job (*)
do {
i++;
} while (i % 1e6 != 0);
if (i == 1e9) {
console.log('Done in ' + (Date.now() - start) + 'ms');
} else {
setTimeout(count); // schedule the new call (**)
}
}
count();
What is a Microtask in JavaScript?
View Answer:
Immediately after every macro task, the engine executes all tasks from the microtask queue before running any other macro tasks or rendering or anything else. All microtasks complete before any other event handling, rendering, or macrotask.
// 3rd: console.logs "timeout" - timeout shows last because it is a macrotask.
setTimeout(() => console.log("timeout"));
// 2nd: console.logs "promise" -
// promise shows second, because .then passes through the microtask queue
Promise.resolve()
.then(() => console.log("promise"));
// 1st: console.logs "code" –
// code shows first because it is a regular synchronous call.
console.log("code");
- Dequeue and run the oldest task from the macrotask queue (e.g., “script”).
- Execute all microtasks
- While the microtask queue is not empty
- Dequeue and run the oldest microtask.
- Render changes, if any.
- If the macrotask queue is empty, wait till a macrotask appears.
- Go to step 1.
What is a Macrotask in JavaScript?
View Answer:
console.log("Start");
setTimeout(() => {
console.log("Inside macrotask");
}, 0);
console.log("End");
In this example, the code will output:
Start
End
Inside macrotask
Even though the setTimeout is set to 0 milliseconds, it still executes asynchronously as a macrotask, allowing other synchronous tasks (like the second console.log) to execute before it.
What is the difference between a microtask and a task (macrotask) in the Event Loop?
View Answer:
Can you explain JavaScript's single-threaded nature?
View Answer:
How does the Event Loop handle asynchronous callbacks?
View Answer:
What are the components of the Event Loop?
View Answer:
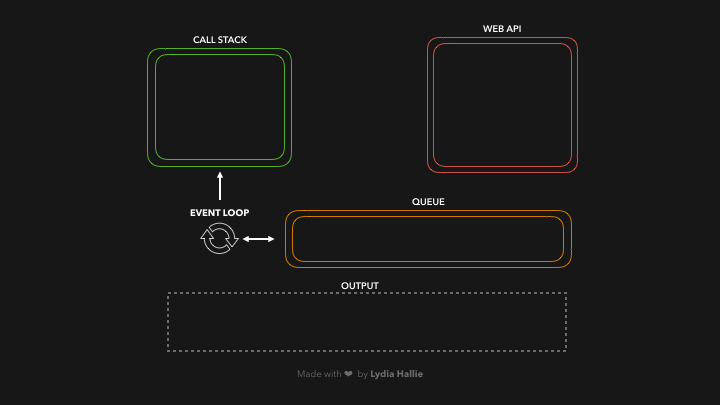
What is the Call Stack in JavaScript?
View Answer:
What is the role of Web APIs in the Event Loop?
View Answer:
Can you explain what the Message Queue is?
View Answer:
What is a zero-delay setTimeout in relation to the Event Loop?
View Answer:
How does Promise resolve order work in the Event Loop?
View Answer:
console.log('Start');
setTimeout(() => console.log('Timeout 1'), 0);
Promise.resolve().then(() => console.log('Promise 1'));
setTimeout(() => console.log('Timeout 2'), 0);
Promise.resolve().then(() => console.log('Promise 2'));
console.log('End');
// The output will be:
// Start
// End
// Promise 1
// Promise 2
// Timeout 1
// Timeout 2
Promises are resolved before timeouts because promise callbacks run in the microtask queue, which is processed before the next run of the macro-task queue (which includes timers).
What is blocking in JavaScript?
View Answer:
What role does the Event Loop play in non-blocking I/O operations?
View Answer:
Can you explain event-driven programming?
View Answer:
document.getElementById('myButton').addEventListener('click', function() {
console.log('Button was clicked!');
});
In this code:
1. An event listener is attached to a button with the id 'myButton'. 2. When the 'click' event is detected (i.e., the user clicks the button), the function is triggered, and an console.log is displayed in the console. This is an example of event-driven programming because the execution of the function is determined by the 'click' event.
How does JavaScript handle concurrent operations?
View Answer:
How are long-running operations generally handled in JavaScript?
View Answer:
Here's an example of a long-running operation, such as a network request, using the Fetch API and async/await syntax in JavaScript.
async function fetchUserData() {
try {
const response = await fetch('https://api.example.com/user');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error:', error);
}
}
fetchUserData();
What happens if the Call Stack is not empty when the Message Queue has tasks?
View Answer:
Can you explain how setImmediate() works?
View Answer:
Here's a simple example of how setImmediate()
works in Node.js.
console.log('Start');
setImmediate(() => {
console.log('setImmediate callback');
});
console.log('End');
// Output:
// Start
// End
// setImmediate callback
In this example, even though the setImmediate()
function call appears before the second console.log()
, its callback function is not run until after all the other code in the current iteration of the event loop has run. Thus, 'End' is logged before 'setImmediate callback'.
What is process.nextTick() in Node.js?
View Answer:
Here's an example of how process.nextTick()
works in Node.js.
console.log('Start');
process.nextTick(() => {
console.log('nextTick callback');
});
console.log('End');
// Output:
// Start
// End
// nextTick callback
In this example, process.nextTick()
schedules the callback function to be invoked in the next iteration of the event loop, but before any other I/O events or timers fire. Despite its placement after the second console.log()
statement, the 'nextTick callback' message is logged after 'End'.